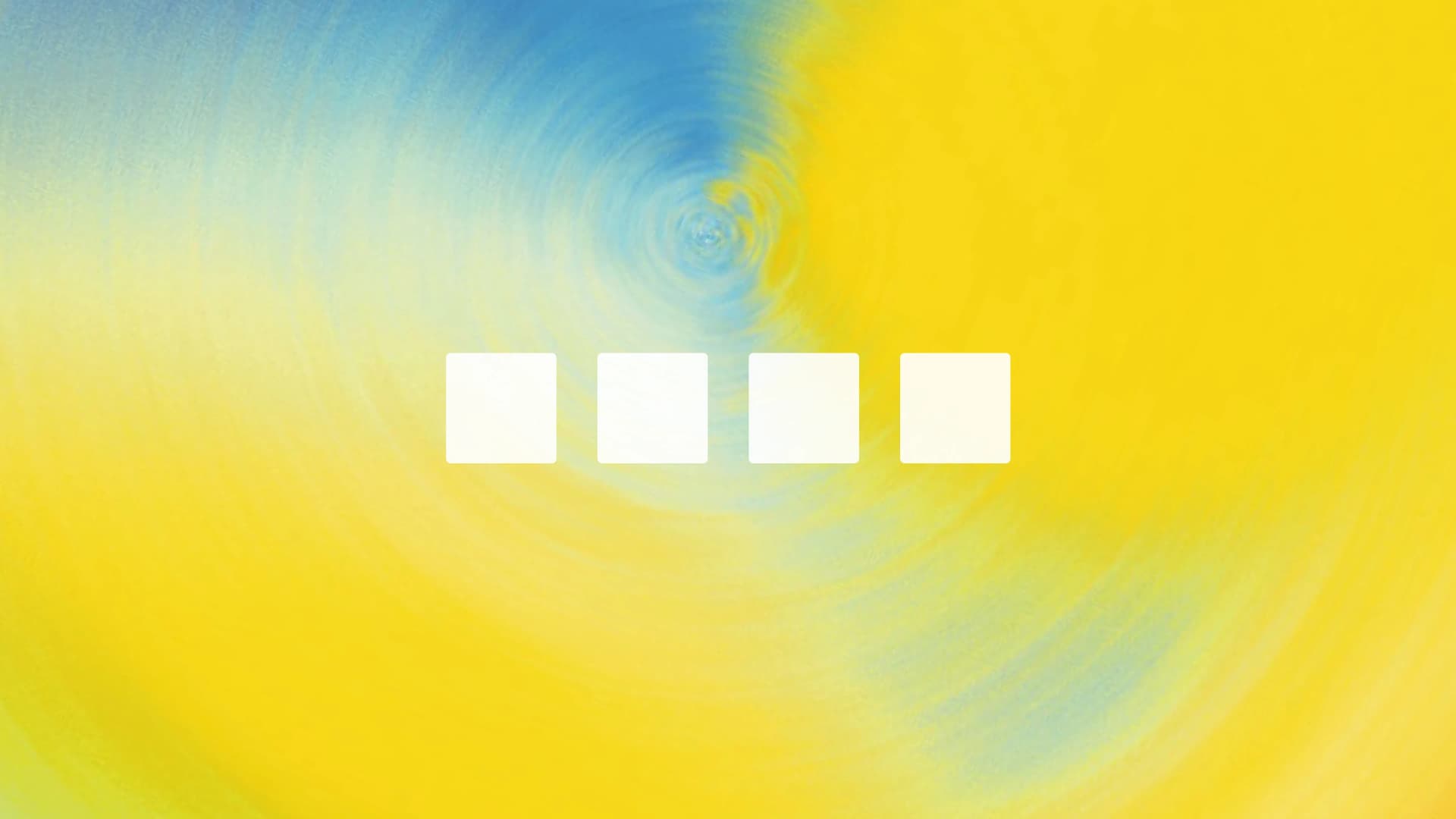
- Introduction
- The Nature of Credit Card Fraud
- Data Collection & Preparation
- Exploratory Data Analysis (EDA)
- Modeling Approaches
- Evaluation Metrics & Performance
- Real-World Challenges & Future Directions
- Conclusion
Introduction
Credit card fraud is a multi-billion-dollar issue that affects consumers, merchants, and financial institutions worldwide. With rising e-commerce transactions and digital payment methods, attackers have become more sophisticated in exploiting vulnerabilities for unauthorized purchases or identity theft. This case study explores how machine learning and data analysis techniques can help detect (and ideally prevent) fraudulent activities in real-time.
Why Fraud Detection Matters
- Financial Losses: Financial institutions and cardholders suffer direct financial hits from fraudulent transactions.
- Reputational Damage: A big breach or high fraud rate can erode customer trust in a bank or merchant.
- Regulatory Compliance: Card issuers and payment networks often enforce strict requirements (like PCI DSS) to protect cardholder data.
- Customer Experience: False positives (flagging genuine transactions as fraud) can anger users, while missed fraud causes financial headaches.
Nature
Common Fraud Schemes
- Stolen Cards: Physical cards stolen and used in person or online.
- Card-Not-Present (CNP) Fraud: Using compromised card details (e.g., from phishing sites, data breaches) to purchase online.
- Identity Theft: Fraudsters open new accounts under someone else’s identity.
- Counterfeit or Cloned Cards: Skimming card details from ATMs or POS terminals and creating duplicates.
Fraud Trends
- Data Breaches: Large-scale hacks of retailer or bank databases give fraudsters access to millions of card numbers.
- Dark Web Marketplaces: Stolen card details or personal information are sold or traded, fueling further attacks.
- Technological Arms Race: As banks adopt better detection methods, criminals shift tactics—highlighting the need for adaptive, data-driven defense.
Data
To detect fraud via machine learning, you need transaction data with details like:
- Amount: The monetary value of the transaction.
- Timestamp: When the transaction occurred.
- Merchant ID: The retailer or site where the purchase was made.
- Location / IP: Geographical or network information.
- Card/Account Information: Possibly hashed or anonymized to protect privacy.
- Fraud Label: Whether the transaction was indeed fraudulent (1) or legitimate (0).
Example Dataset Credit Card Fraud from Kaggle
- High Class Imbalance: Often <1% of transactions are fraudulent.
- Privacy & Security: Real-world data is sensitive, meaning it’s usually masked, limited, or anonymized.
Data Cleaning
- Check Missing Values: Typically, real fraud data has few to no missing entries, but always confirm.
- Duplicate Transactions: Remove or investigate duplicates—sometimes repeated records can be a sign of malicious or test transactions.
- Derived Features: You might add extra columns, e.g., “time since last transaction,” “transaction frequency per user,” or “spending pattern at certain hours.”
EDA
Distribution of the Target Variable
import matplotlib.pyplot as plt
import seaborn as sns
# Suppose we have a DataFrame 'df' with 'Class' indicating fraud or not
sns.countplot(x='Class', data=df)
plt.title("Distribution of Fraud (1) vs. Legitimate (0)")
plt.show()
- Usually, ~99.8% transactions are legitimate and only ~0.2% are fraud. This imbalance means naive methods (like always predicting “legitimate”) yield high accuracy but miss actual fraud.
Correlation & Feature Relationships
- If your data has raw features (like transaction amount, time, location), you can plot correlation matrices or scatterplots to see if certain variables (e.g., large amounts, unusual times) correlate strongly with fraud.
Time & Amount Insights
- Time-based: Do frauds spike at certain times of day or day of the week?
- Amount-based: Are fraudulent transactions typically large or small amounts?
Real insight: Some fraud rings do test small micro-transactions first, ensuring the card is active before big purchases.
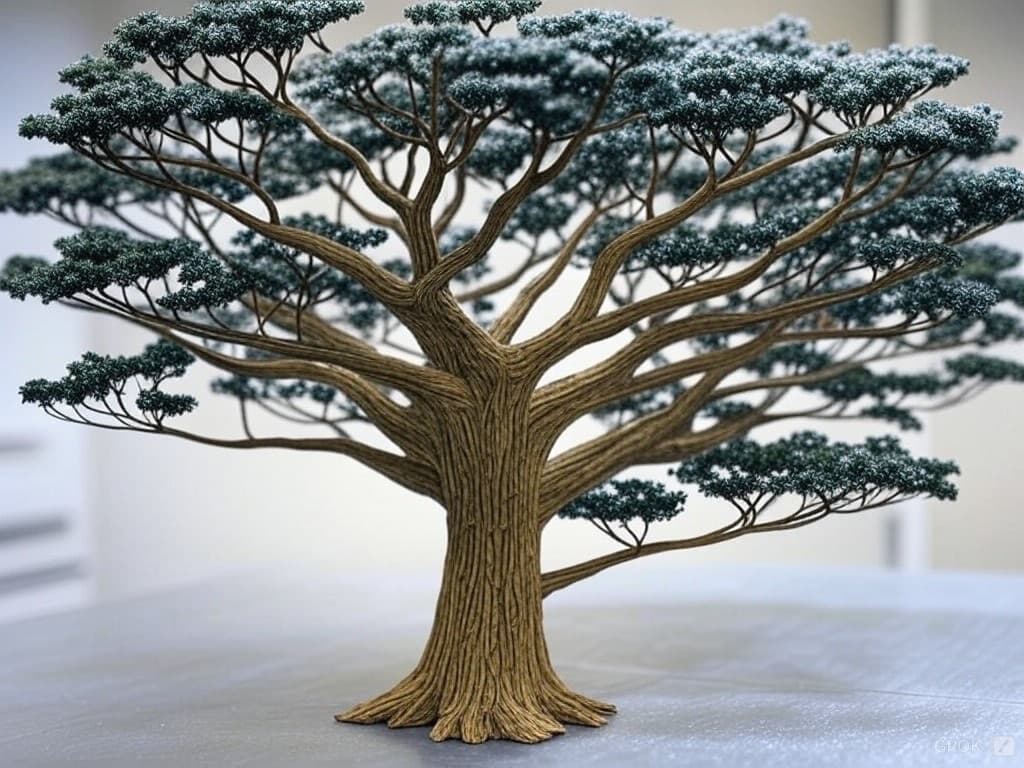
Modeling Approaches
Data Imbalance Solutions
-
Because only a tiny fraction of transactions are fraud, standard classifiers might ignore the minority class. Potential solutions:
-
Undersampling: Randomly select a subset of legitimate transactions to match the number of fraud cases.
-
Oversampling / SMOTE: Synthetically create or replicate fraud cases to increase the minority class.
-
Class Weighting: Adjust the training loss so errors on fraud cases are penalized more heavily.
Classification Algorithms
-
Logistic Regression
- Pros: Interpretable coefficients, easy to implement.
- Cons: May not capture complex, non-linear relationships unless properly feature-engineered.
-
Tree-Based Methods (Random Forest, XGBoost)
- Pros: Often perform well on tabular data; handle non-linearities.
- Cons: Large ensembles can be memory- or compute-intensive, but typically robust for fraud detection.
-
Neural Networks
- Pros: Potentially high predictive power with enough data.
- Cons: Harder to interpret; can be overkill unless you have a massive dataset and complex patterns.
-
Ensemble Methods (Voting, Stacking)
- Pros: Combine multiple models for better performance.
- Cons: Increased complexity and potential overfitting if not tuned properly.
Using a Random Forest
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import classification_report, confusion_matrix
# Suppose df['Class'] is the target, X are the features
X = df.drop('Class', axis=1)
y = df['Class']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42, stratify=y)
# Random Forest with class_weight='balanced_subsample' to handle imbalance
rf = RandomForestClassifier(n_estimators=100, class_weight='balanced_subsample', random_state=42)
rf.fit(X_train, y_train)
y_pred = rf.predict(X_test)
print(confusion_matrix(y_test, y_pred))
print(classification_report(y_test, y_pred))
If today were the last day of my life, would I want to do what I am about to do today? – Steve Jobs
Evaluation Metrics & Performance
Beyond Accuracy Accuracy is misleading with highly imbalanced data (99.8% legit vs. 0.2% fraud). If you predict everything as “legit,” you get 99.8% accuracy but miss all fraud.
- Precision (Fraud): Of transactions predicted as fraud, how many are truly fraud?
- Recall (Fraud): Of all actual fraud transactions, how many did we catch (true positives)?
- F1 Score: Harmonic mean of precision and recall, balancing both.
ROC & AUC
- ROC Curve: Plots the true positive rate vs. false positive rate at different thresholds.
- AUC: The area under the ROC curve. A good model has AUC close to 1.0; random guessing yields 0.5.
Precision-Recall Curve
- More appropriate for highly skewed classes: If you want to catch as many frauds as possible (high recall) but keep an acceptable false positive rate (precision), you might adjust thresholds or focus on the PR curve.
Real-World Challenges & Future Directions
- Evolving Fraud Tactics: Attackers change strategies quickly, requiring continuous model retraining or online learning. Latency & Real-Time Constraints: Fraud detection systems often must run near real-time on streaming data.
- Cost of Misclassification: Missing a fraud can be expensive, but flagging too many legitimate transactions also hurts user experience. Some businesses adopt cost-sensitive approaches, weighting false negatives more.
- Explainability: In regulated industries, you must often explain why a transaction is flagged. Decision trees, SHAP, or feature importance tools can help.
- Scalability: Large banks handle millions of transactions daily—distributed computing and efficient data pipelines become critical. The Role of Feature Engineering
- Geolocation: Deviations from usual user location can signal risk.
- Device Fingerprinting: If a new device is used or there’s an unusual user-agent string, it can indicate potential fraud.
- Aggregations: Summaries like “number of transactions in the past hour” or “total amount spent in 24 hours” highlight suspicious bursts of activity. Advanced Methods
- Deep Learning: LSTM networks on transaction sequences.
- Graph-Based Approaches: Model user/card relationships as a graph to catch suspicious networks.
- Anomaly Detection: Real-time anomaly scoring via sliding windows.
Dropped ML Model case study checkout presentation @cdacindia youtube.com/watch?v=Dcc4-i…
It is not the strongest of the species that survive, nor the most intelligent, but the one most responsive to change. – Charles Darwin
Kaggle Code is a clean, simple, and fast learning explained code